This post i will show you , get user location details using his IP. Here i will use Maxmind. Which is best among all others. Every other package is also using Maxmind.
Step 1. Sign Up to Maxmind.
Step 2. Download Database from Maxmind.
Step 3. Install Package through Composer.
Step 4. Setup the Maxmind in Laravel Project.
Step 5. Create Controller and get all users location.
Step 1. Sign Up to Maxmind:
Visit this link and register with your email.
https://www.maxmind.com/en/accounts/522958/geoip/downloads
Step 2. Download Database from Maxmind:
Scroll down and download as like below image.
Step 3. Install Package through Composer:
Before going to install package , please install laravel using the below command.
composer create-project --prefer-dist laravel/laravel laravel9GeoIp
Note: laravel9GeoIp is my project name. You can give our own.
Now install package .
composer require geoip2/geoip2
Step 4. Setup the Maxmind in Laravel Project:
A. Create a new directory inside the app/ , With name “Traits”. For reference check below image.
B. Create a new file inside the app/Traits/ , With name “MaxMindGeoIP2Trait.php“.
C. MaxMindGeoIP2Trait.php Open the file and put the below code.
<?php namespace App\Traits; use Exception; use GeoIp2\Database\Reader; use Illuminate\Support\Str; use MaxMind\Db\InvalidDatabaseException; use GeoIp2\Exception\AddressNotFoundException; trait MaxMindGeoIP2Trait { protected function getGeoIp2($IP) { $geoDataArray = array(); try { if(filter_var($IP, FILTER_VALIDATE_IP)) { $reader = new Reader(base_path().'/database/MaxMindGeoIP2/GeoIP2-City.mmdb'); $record = $reader->city($IP); $geoDataArray = array( 'cityName' => $record->city->name, 'latitude' => $record->location->latitude, 'longitude' => $record->location->longitude, 'timeZone' => $record->location->timeZone, 'zipCode' => $record->postal->code, 'regionName' => $record->mostSpecificSubdivision->name, 'countryName' => $record->country->name, 'countryCode' => strtoupper(Str::of($record->country->isoCode)->replace(array(' ', '\\', '/'), array('', '', ''))->trim()->__toString()), 'ipAddress' => $record->traits->ipAddress, ); } } catch (AddressNotFoundException $e) { } catch (InvalidDatabaseException $e) { } catch (Exception $e) { } return $geoDataArray; } } ?>
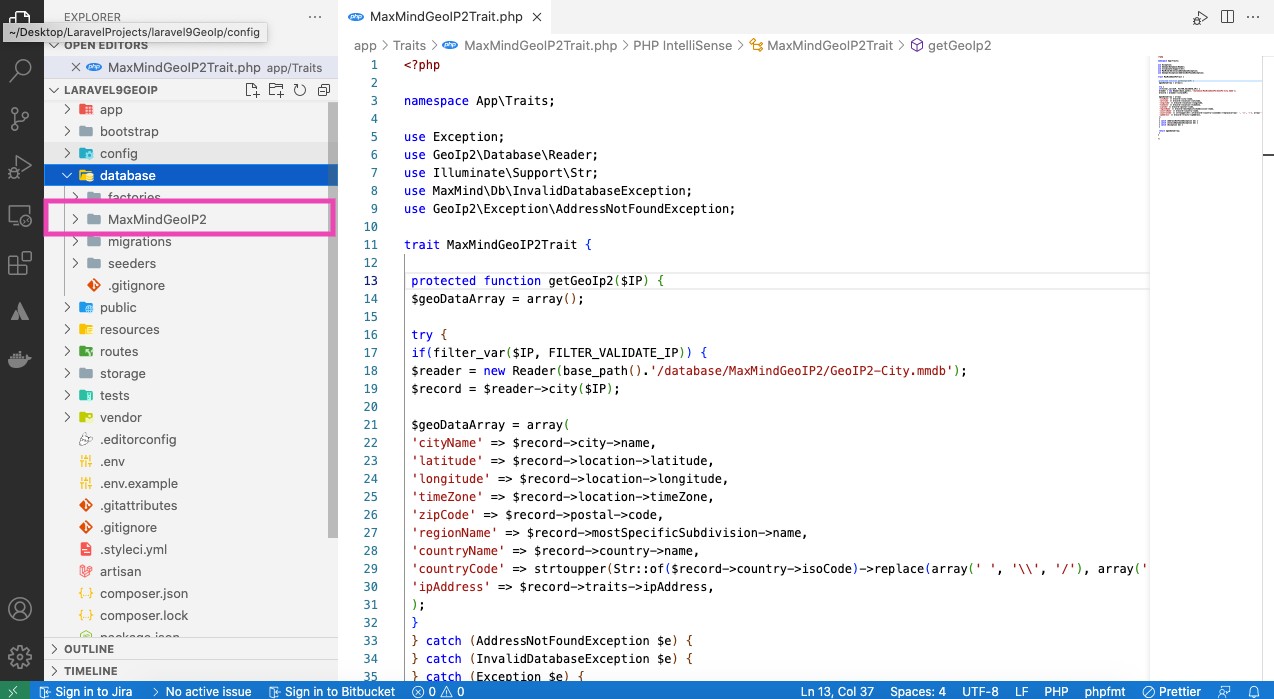
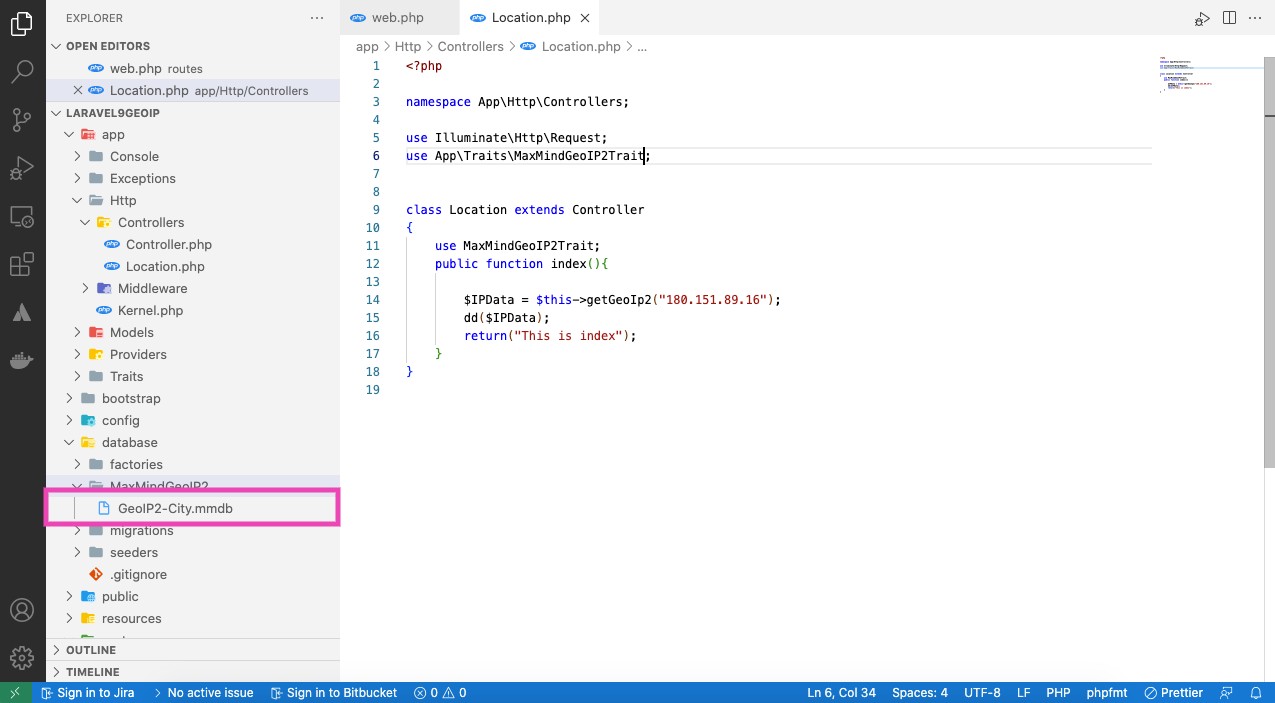
Step 5. Create Controller and get all users location.
Create a controller, I am creating with the name of “Location“.
php artisan make:controller Location
Open the file and put the below code .
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Traits\MaxMindGeoIP2Trait; class Location extends Controller { use MaxMindGeoIP2Trait; public function index(){ $IPData = $this->getGeoIp2("180.151.89.16"); dd($IPData); return("This is index"); } }
Also define your route. Open the routes/web.php
<?php use Illuminate\Support\Facades\Route; use App\Http\Controllers\Location; Route::get('/', function () { return view('welcome'); }); Route::get("location",[Location::class,"index"]);
Run this project and check, You will find like below, If you have any problem please comment below.
Happy Coding….